Welcome back everyone 👋 and a heartfelt thank you to all new subscribers who joined in the past week!
This is the 90th issue of the Gorilla Newsletter—a weekly online publication that sums up everything noteworthy from the past week in generative art, creative coding, tech, and AI.
If it's your first time here, we've also got a Discord server where we nerd out about all sorts of genart and tech things — if you want to connect with other readers of the newsletter, come and say hi: here's an invite link!
Before we get into this week's roundup of creative tech shenanigans, let's talk about plotters 👇
Getting into Pen Plotting
As the banner of this week's issue might already have revealed — I got a plotter!
It arrived this past Monday actually, just a couple of hours after the previous issue of the newsletter had gone out. I didn't waste much time, quickly set it up, and had it trace out some sketches.
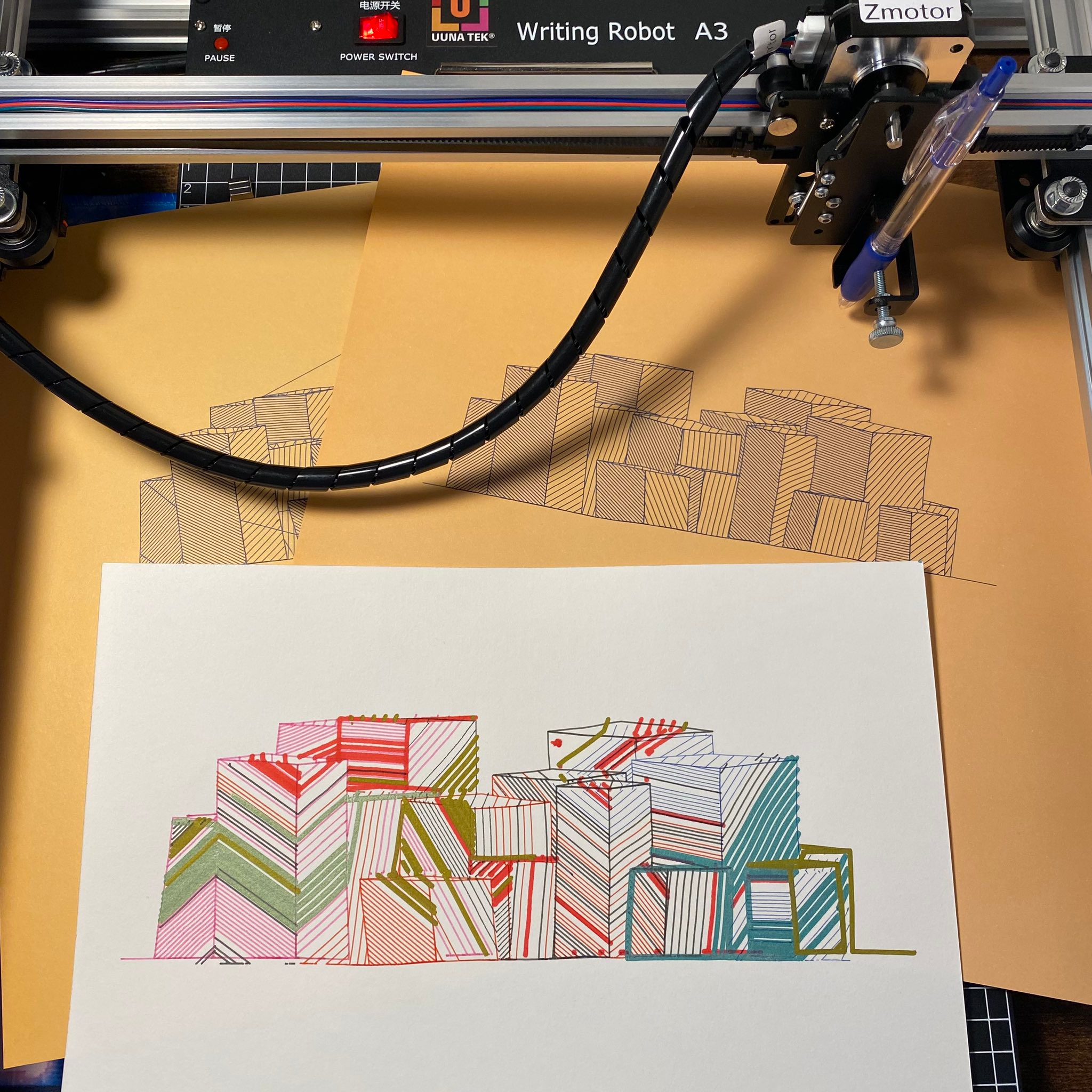
I've enjoyed the process tremendously — not only because it's quite meditative to watch the plotter slowly bring physical instances of my sketches to life, but also because it's brought up some interesting new technical challenges that I had to figure out.
Luckily there's tools like Zenozeng's SVG runtime for P5, that I've previously explored way back in 2022, as well as Golan Levin's newer p5.plotSvg, both of which make it quite straightforward to turn P5 outputs into plottable SVGs.
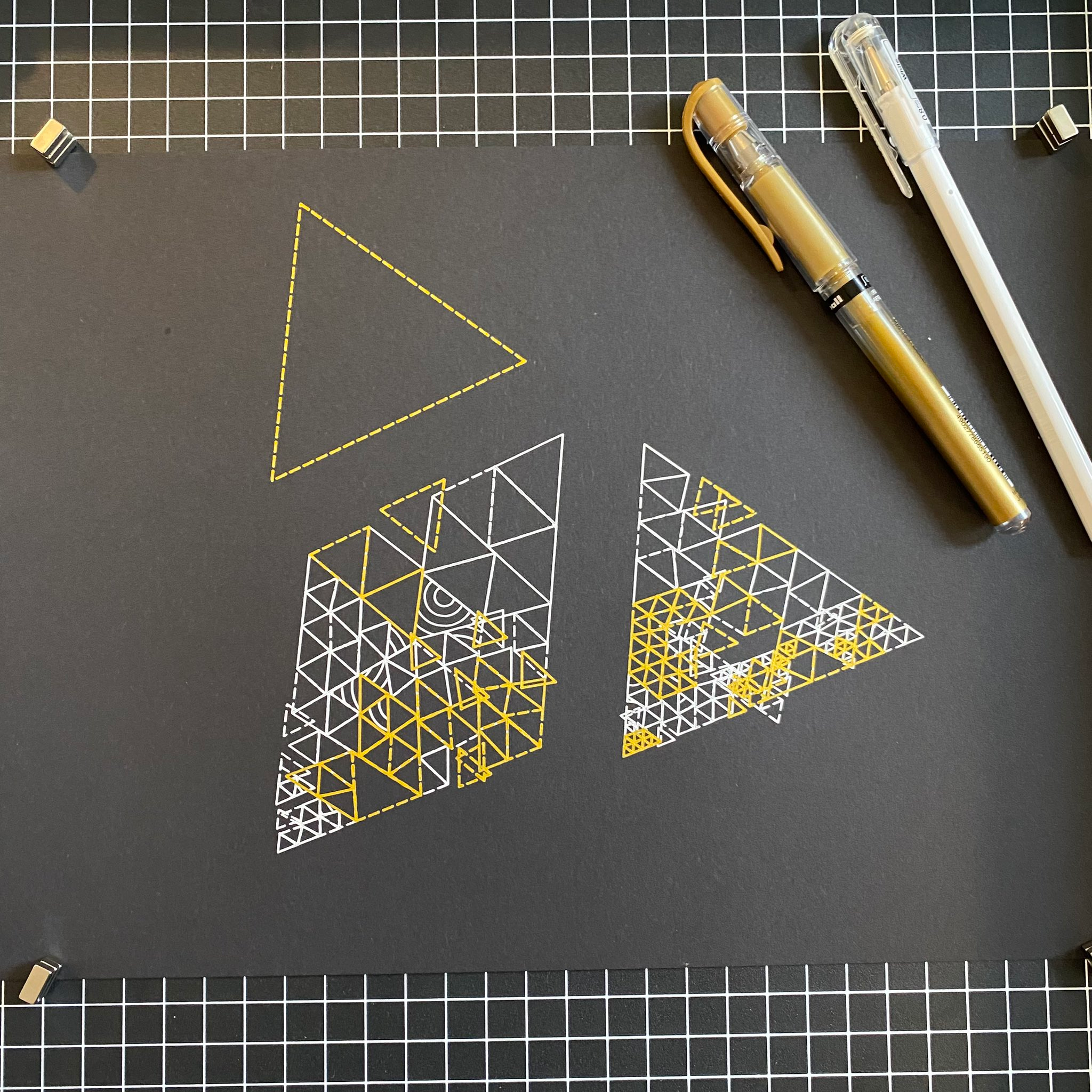
With the plotter you also need to start thinking a bit more about what works well on paper, rather that what is possible digitally (everything) — which is honestly a welcome restriction that I'm happy to embrace.
I also already discovered some other useful tools, like the axidraw hidden line removal plugin for inkscape that's quite handy when you have many overlapping shapes, as well as the svgo command line tool, that will not only trim down your svg files but also reorder the paths so that the plotter doesn't waste time jumping around back and forth.
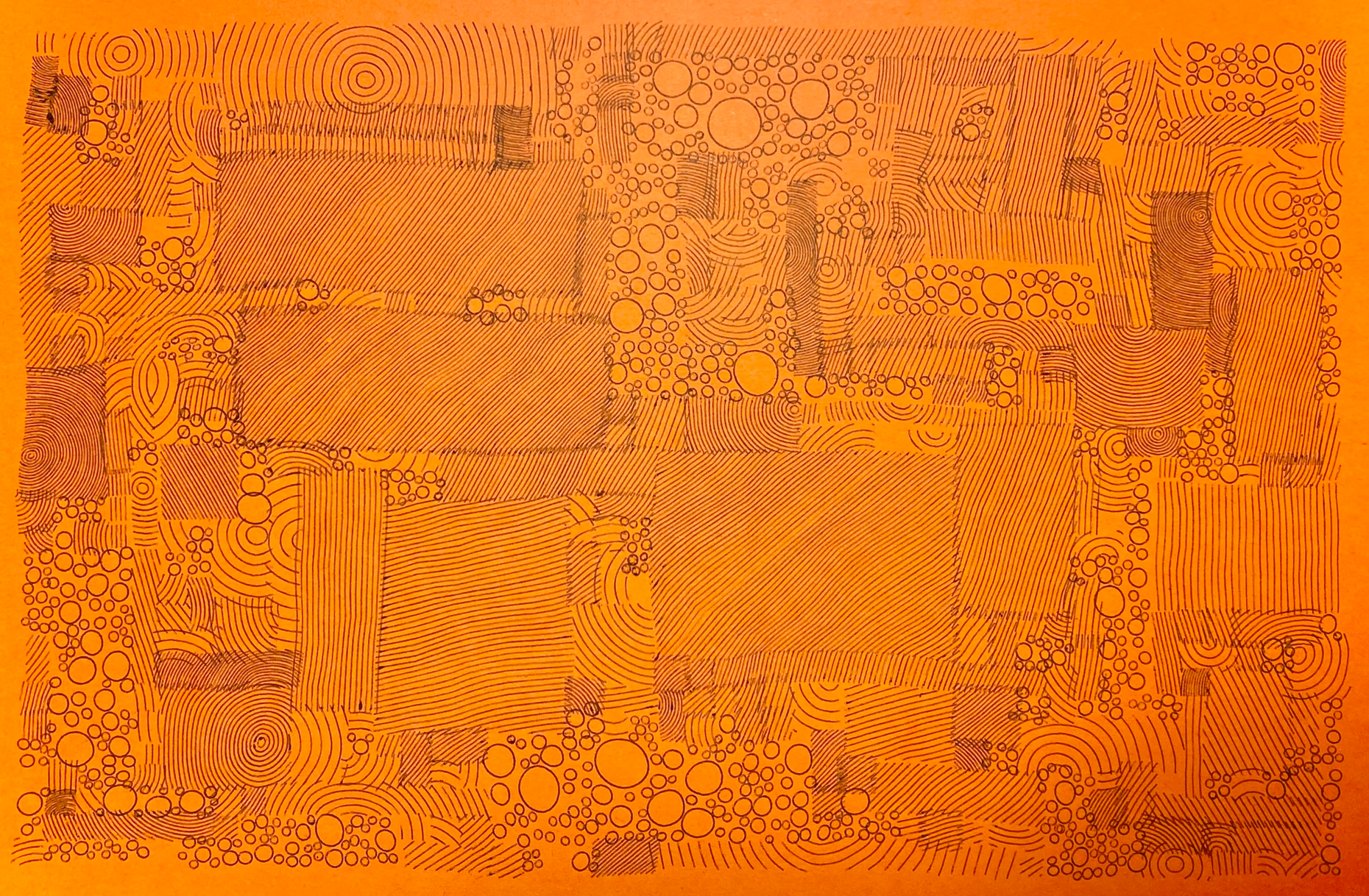
A gigantic shout-out to Dan Catt here, and all the content he's made around pen plotting — it's been a tremendous help! Check out his YouTube here.
I'll have more updates/pics to share next week — feel like I'm slowly getting the hang of it here. One issue I'm running into right now though, is how to not spend all of my money on pens and paper 😆
That said — cue the news 👇
All the Generative Things
1 — Learn Shader Programming with Rick and Morty: kicking off this week with a Rick and Morty themed programming tutorial — Daniel Hooper shows us the ropes of shader programming by recreating Rick's recognizable features in 240 lines of GLSL code.
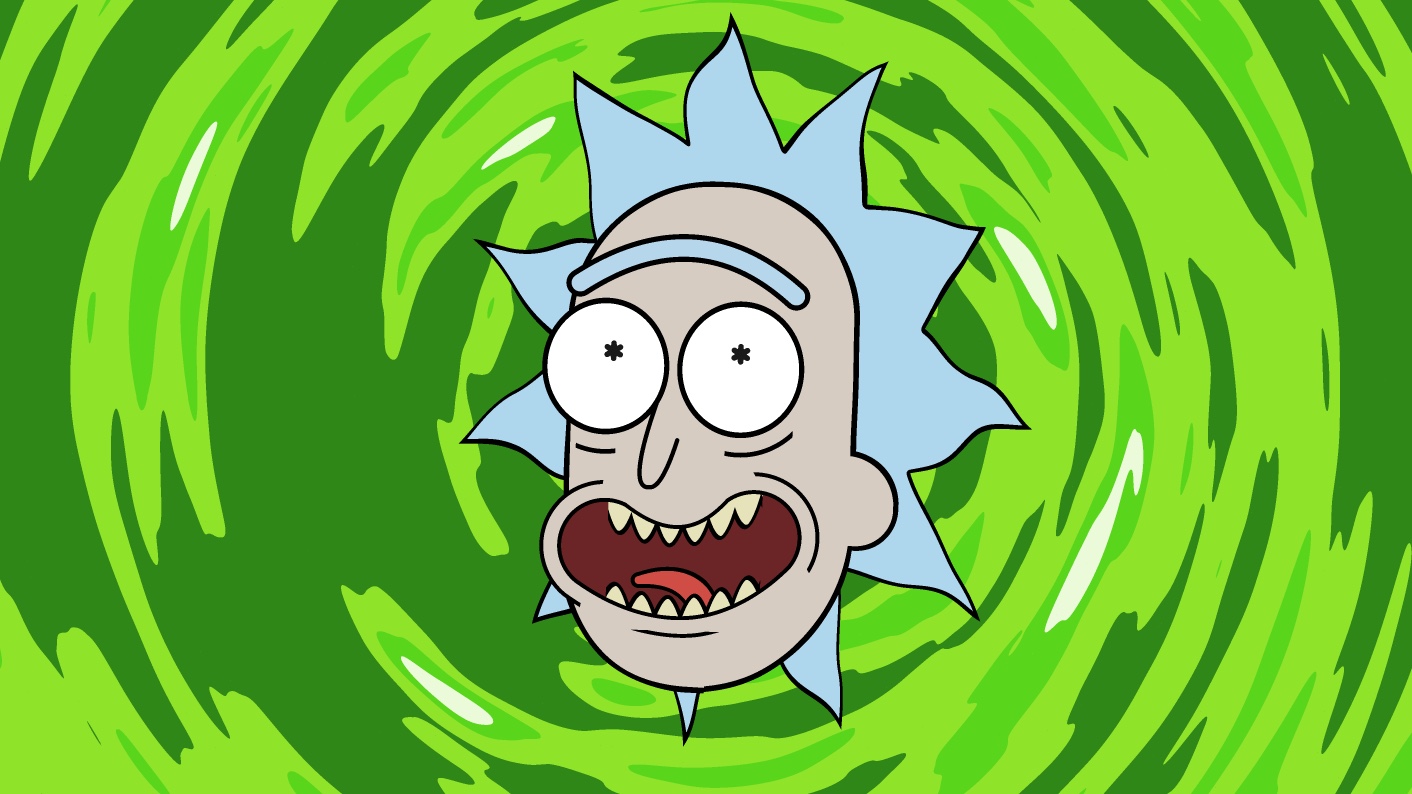
The tutorial starts by explaining SDFs to create basic shapes that'll make up individual parts of Rick's face, and then shows how to combine them into more interesting composite shapes by computing their union — simply with the min()
function. This is a trick that I sorta discovered by myself before, while doing my silly little non-shader SDF experiments. Okay, what the heck — here's a mini tutorial 😆
With P5JS we can simulate a dumb shader by looping over all the pixels of the canvas, and then setting the color of specific pixels if they falls inside a certain region we defined — in the following example we're simply drawing two circles:
let d1 = dist(x,y,C1.x,C1.y) - C1.r
if(d1 < 0) buff.set(x,y, color(255,0,0))
let d2 = dist(x,y,C2.x,C2.y) - C2.r
if(d2 < 0) buff.set(x,y, color(0,0,255))
You get something like this:
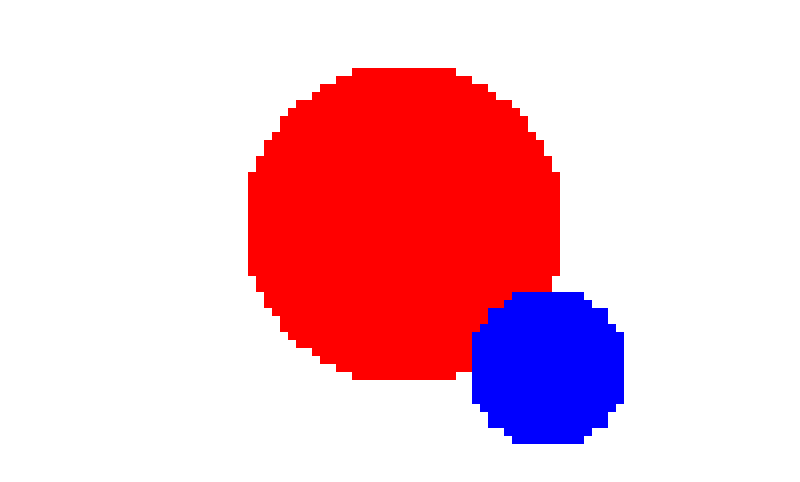
There's a better way to do this though, without doing two separate if statements — yes, you could just do a logic or ||
— but you could also combine the two regions as one by getting the minimum distance, as such:
let d = min(d1,d2)
if(d < 0) set(x,y, color(255,0,0))
if(abs(d) < .5) set(x,y, color(0,0,0)) // colors the outline in black
Then you'd get something like the below render, where it also becomes really easy to set the color of the region's outline:
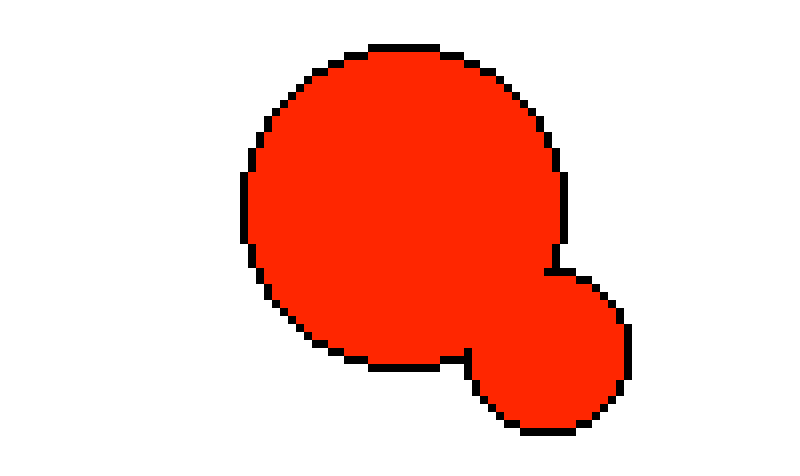
Towards the end of the tutorial Daniel also shows us how to export the animation as a video — and that simply with bash script!
2 — How to not "optimize" GPU conditionals: while we're already talking about GLSL, here's an important PSA from Inigo Quilez — he posts about a common misconception that's often erroneously advised to be a math trick for optimizing conditional moves on the GPU.
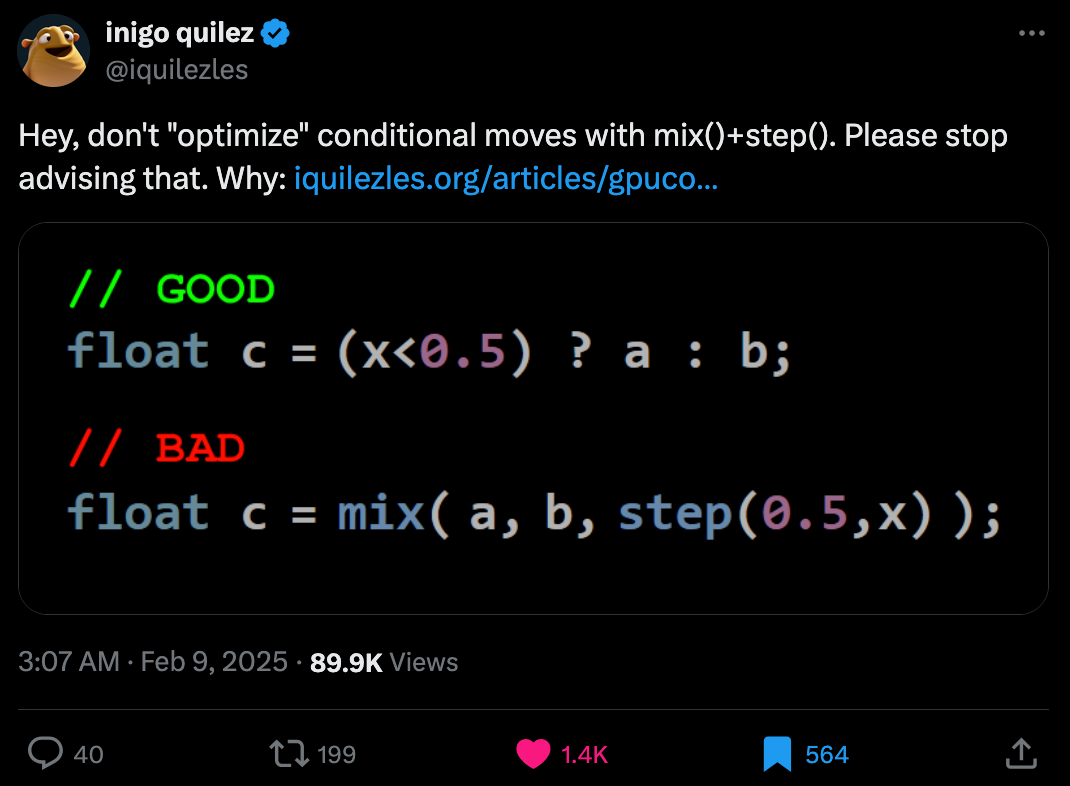
I had to do a little bit of research to understand this, because I'm not by any means an expert; but in essence, GPUs run many threads in groups called warps, all of which follow the same instructions at the same time. When an if
statement causes some threads to take one path and others to take a different one, the GPU has to run these paths separately, one after the other. This obviously slows things down because while one set of threads is working, the others have to wait — this problem is known as warp divergence (which sounds like something straight out of Star Trek).
The issue that Inigo Quilez points out is that some people mistakenly believe that using ternary operators (? :
) or if
statements in shader code introduce this warp divergence. In cases where the condition simply selects between values (the code snippet he demonstrates in his post), the GPU does not actually branch — it simply performs a conditional move (select), which is an efficient instruction that does not disrupt execution flow.
The misguided "optimization" replaces the ternary-based selection with arithmetic masking (step()
functions and multiplications), thinking it avoids the GPU from branching. But in reality, this approach actually introduces unnecessary calculations, making the shader slower. Inigo Quilez confirms this by inspecting the compiled GPU machine code, showing that the original ternary-based code doesn't branch, and that the so-called optimization is both unnecessary and counterproductive.
3 — Simulating Water over Terrain: Lisyarus shows us how to create realistic water behavior for top-down, grid-based games in which the terrain can be dynamically modified. He introduces a method called "virtual pipes" where neighboring water cells in the game are connected by imaginary pipes to simulate the flow of water between them.
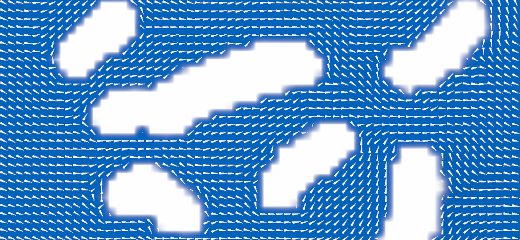
In a nutshell, it's basically a simulation based on a simplified version of the Navier-Stokes equations, also known as the shallow-water equations (SWE). Instead of working in 3D, things are flattened down to 2D, averaging the water's behavior along the vertical direction. This requires the use of a staggered grid, where the water's height is stored inside the grid cells, whereas water flow is stored along the edges between cells.
In a very oversimplified manner of explaining things, water moving between cells depends on the difference in elevation, and the amount of water held by each cell. Naturally the water will flow from higher to lower positions, and the quantity will also balance itself out — Lysiarus shares the technical details in the tutorial and also provides a WebGPU implementation here.
Noteworthy here is that this same approach is used in a game called Timberborn, a post-apocalyptic beaver themed city-building game:
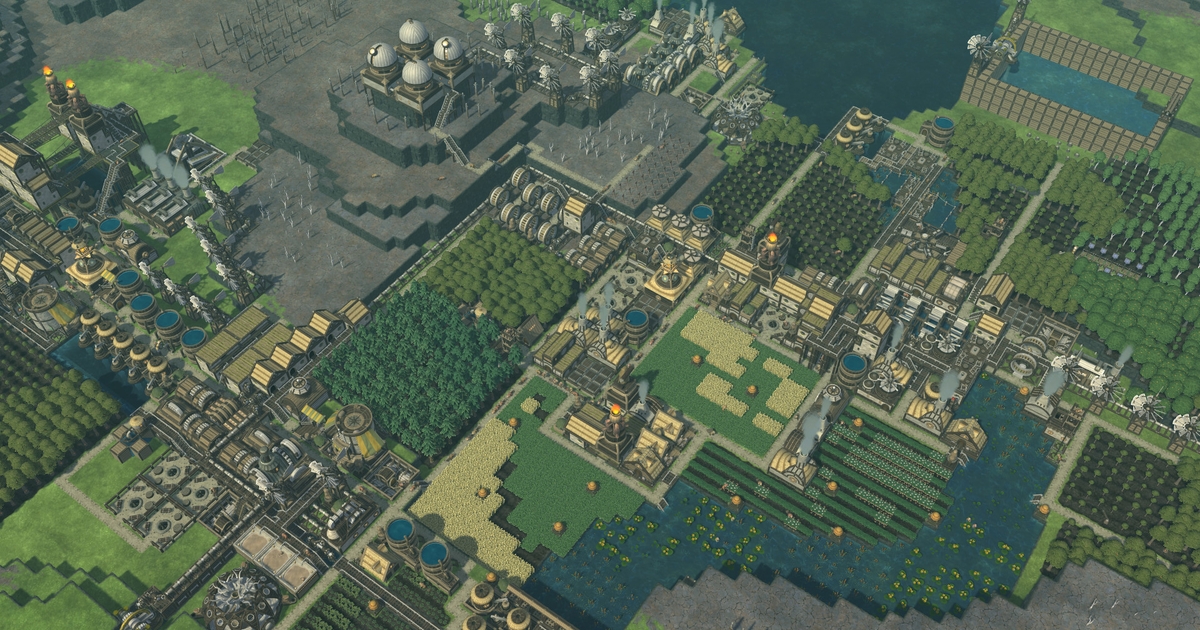
4 — A Year of Learning WebGPU: if you've been meaning to get into WebGPU, Javi Agenjo shares notes he took over the course of a year of learning — in 96 slides he provides a complete introduction to WebGL's successor.
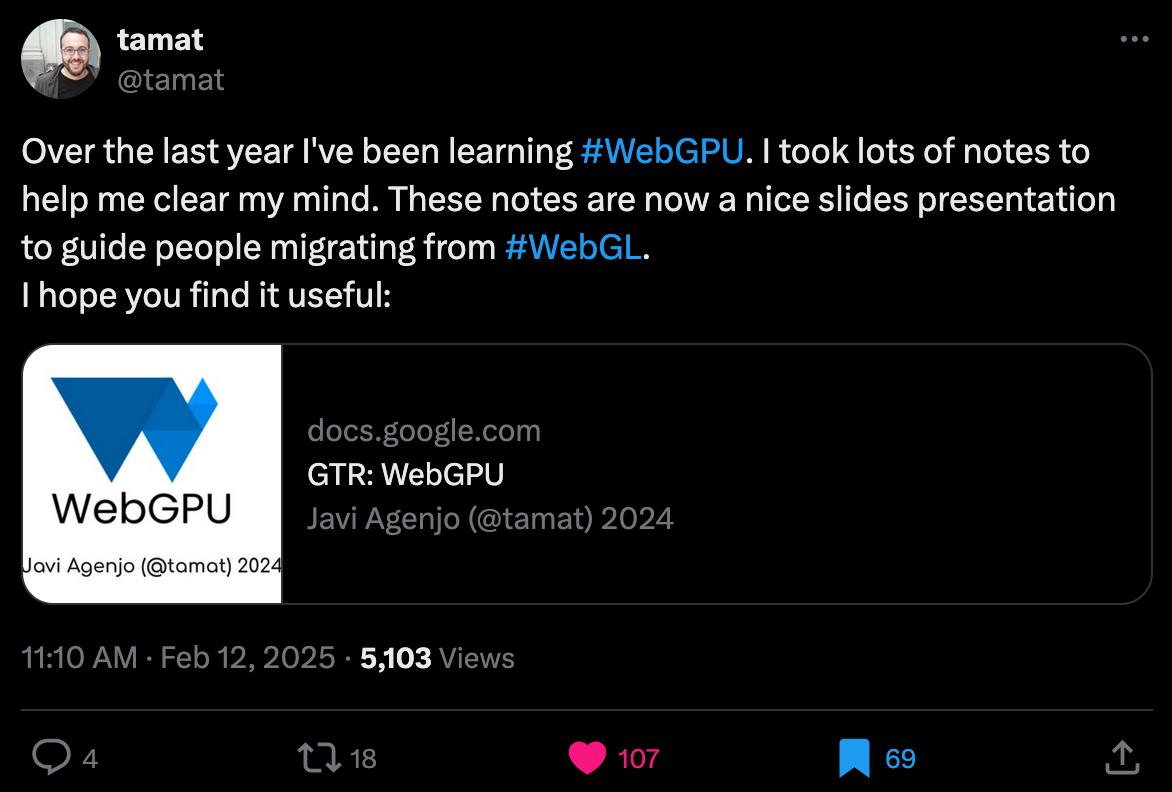
5 — Visualizing Data is an Art: Shri Khalpada argues that successful data visualization isn't just about technical accuracy or perceptual precision, but should also provide new perspectives on the data, spark curiosity, and even tell a story.
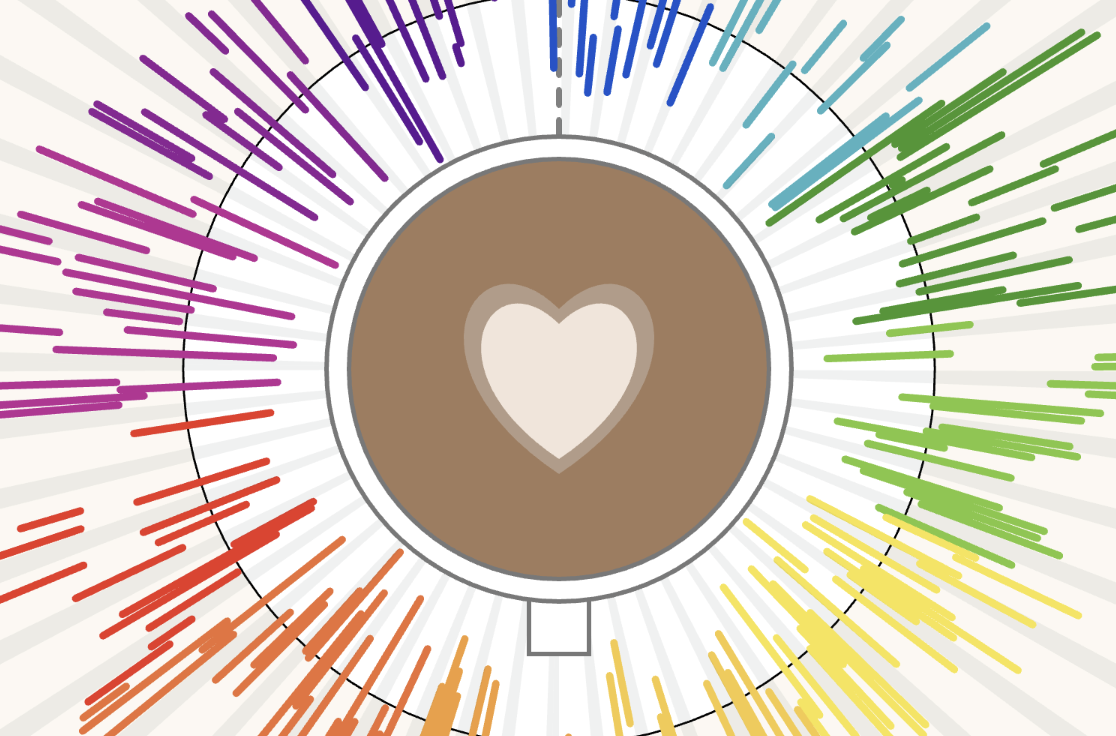
Tech & Web Dev
1 — Thinkserver: my web-based coding environment: after having heavily used Glitch for creating projects, a platform where devs can create and share websites with minimal setup, Christian Lawson was inspired to build his own web-based coding environment tailored towards his needs — he details how it works in an blog post of his:
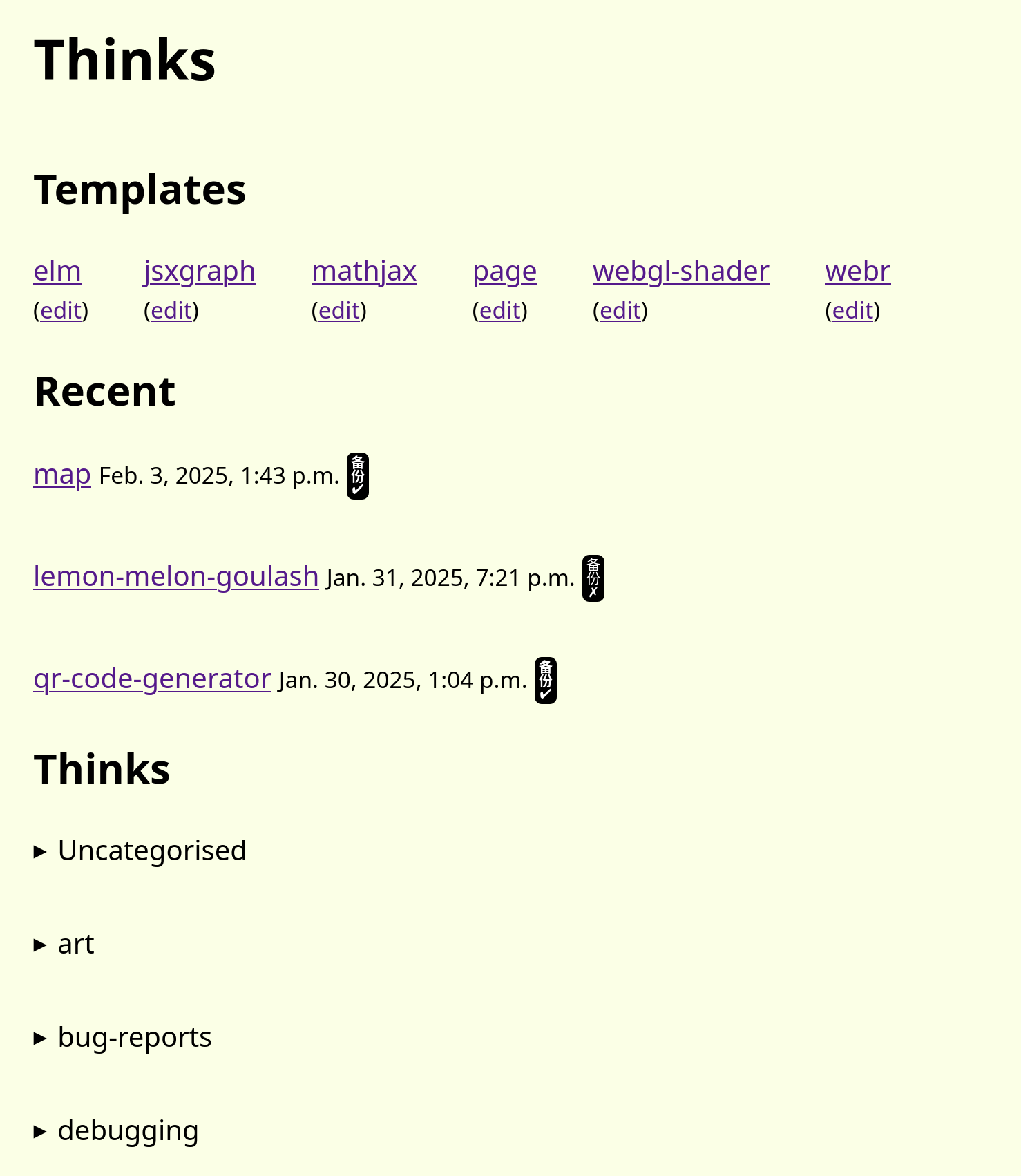
After a year of using it and creating around 80 small projects, he shares the code for it over on his Forgejo instance. What's worth pointing out is that it strongly resonated with the folks over on Hacker News, with many sharing stories about their own custom coding environments to build small useful tools:
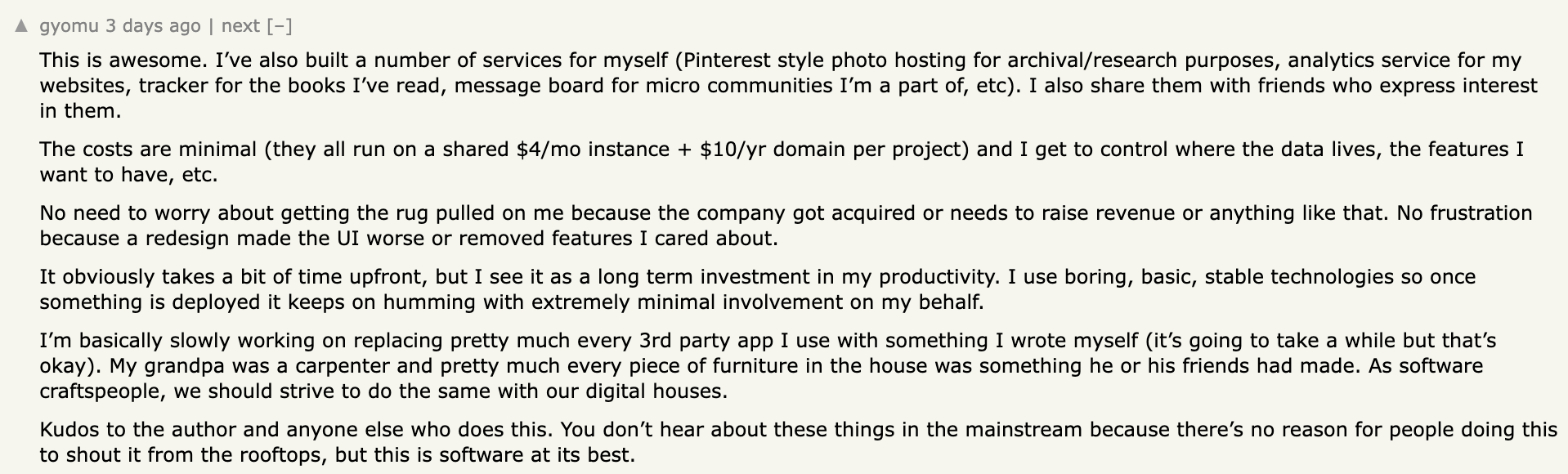
2 — The hardest working font in Manhattan: Gorton is an overlooked font that's seemingly almost everywhere you look in New York City — Marcin Wichary shares how he discovered and tracked it down while photographing different corners of NYC, and lets us in on the fascinating backstory of the font:
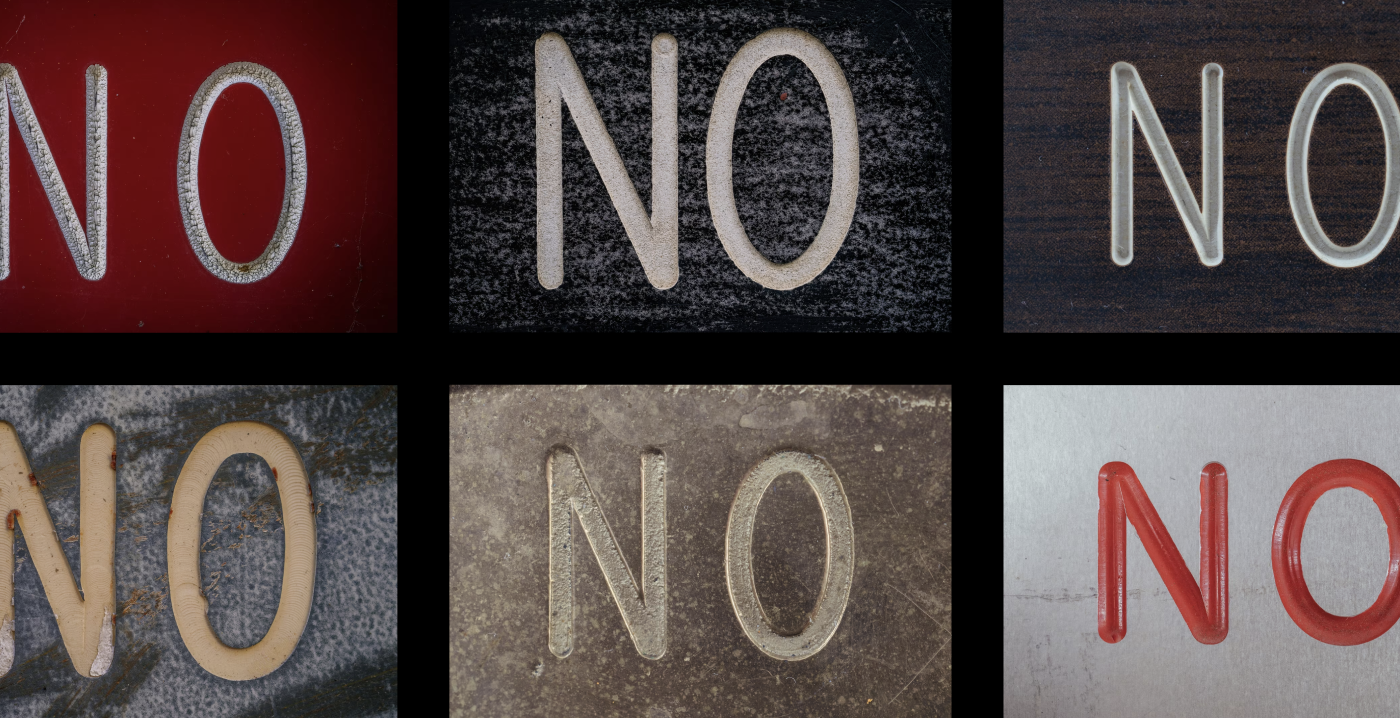
Originally created for engraving machines in the early 1900s, Gorton found its way onto everything from subway signs and machinery to space equipment — Gorton's mechanical design and durability made it perfect for signs and labels that needed to last, which is why you find it in places like nuclear facilities, and even the Apollo moon missions.
Can we also take a moment to appreciate how incredibly stylish Marcin's website is?
3 — Leaking the email of any YouTube user for 10 grand: security researcher brutecat discovered that blocking someone on YouTube exposes their linked Gaia ID — a unique internal backend identifier used by Google to track user accounts across its services. By itself this ID isn't sufficient to reveal someone's email address, but they didn't stop there however, and figured that the ID can be fed into the endpoints of older Google product, like the Pixel Recorder app, to resolve into an email address.
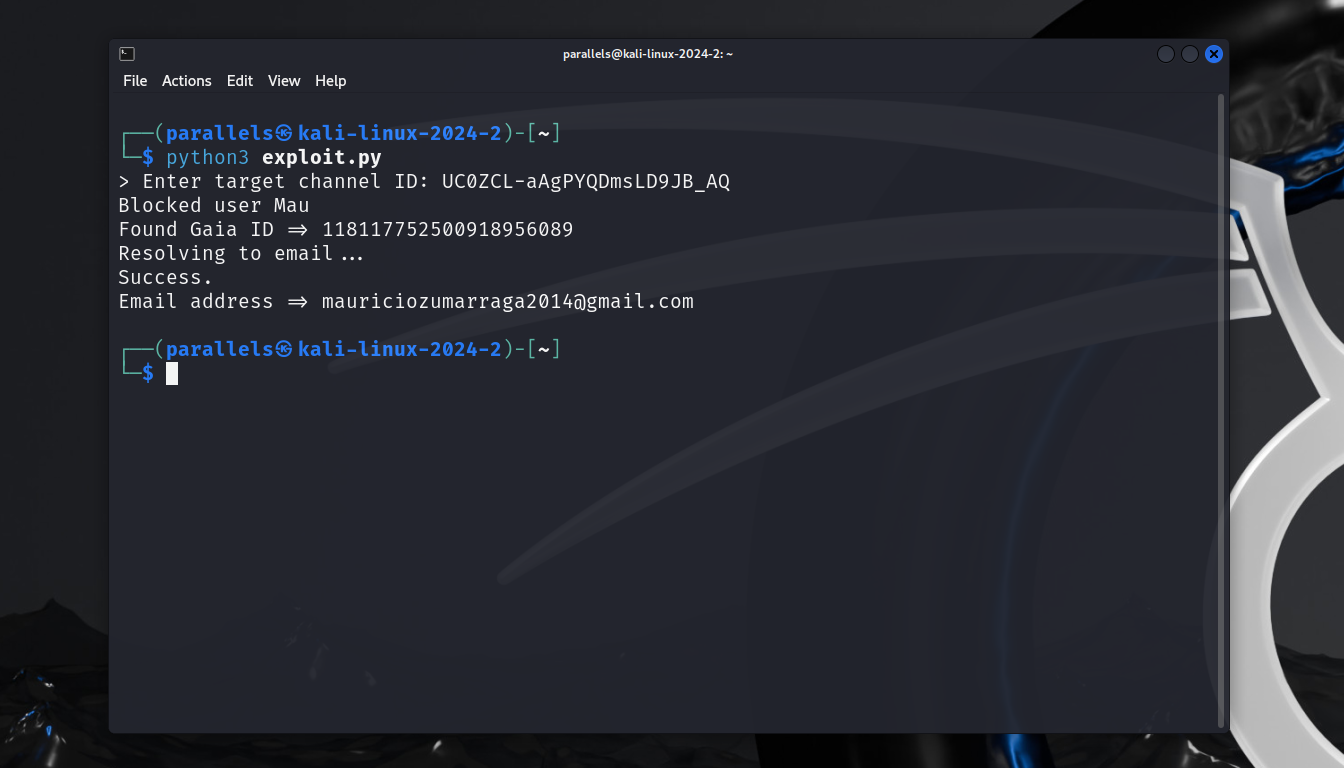
4 — How Apple Designs Virtual Knobs: an older article, that was apparently written in 2012, but only published in 2022 — Jacob Herr explains the clever design behind Garageband’s virtual knobs and what goes on behind the scenes for them to respond to different touch gestures like spinning and sliding. Jacob's investigation culminated in the development of Knob.js, a JavaScript library that mimics this knob behavior.
AI Corner
1 — The End of Programming as We Know It: expert Tim O’Reilly argues that it's unlikely programmers will lose their jobs anytime soon, and rather than rendering the profession obsolete, it's simply a fundamental shift in programming overall — O'Reilly echoes many of the articles I've shared on the newsletter already on this topic. I believe that the fearmongering is generally just a reaction to how quickly things are evolving, but not actually a reflection of what is happening. The reaction to the paradigm shift AI introduces, isn't so different from the times when new "revolutionary" technologies were introduced — each time being proclaimed as another "End of Programming".
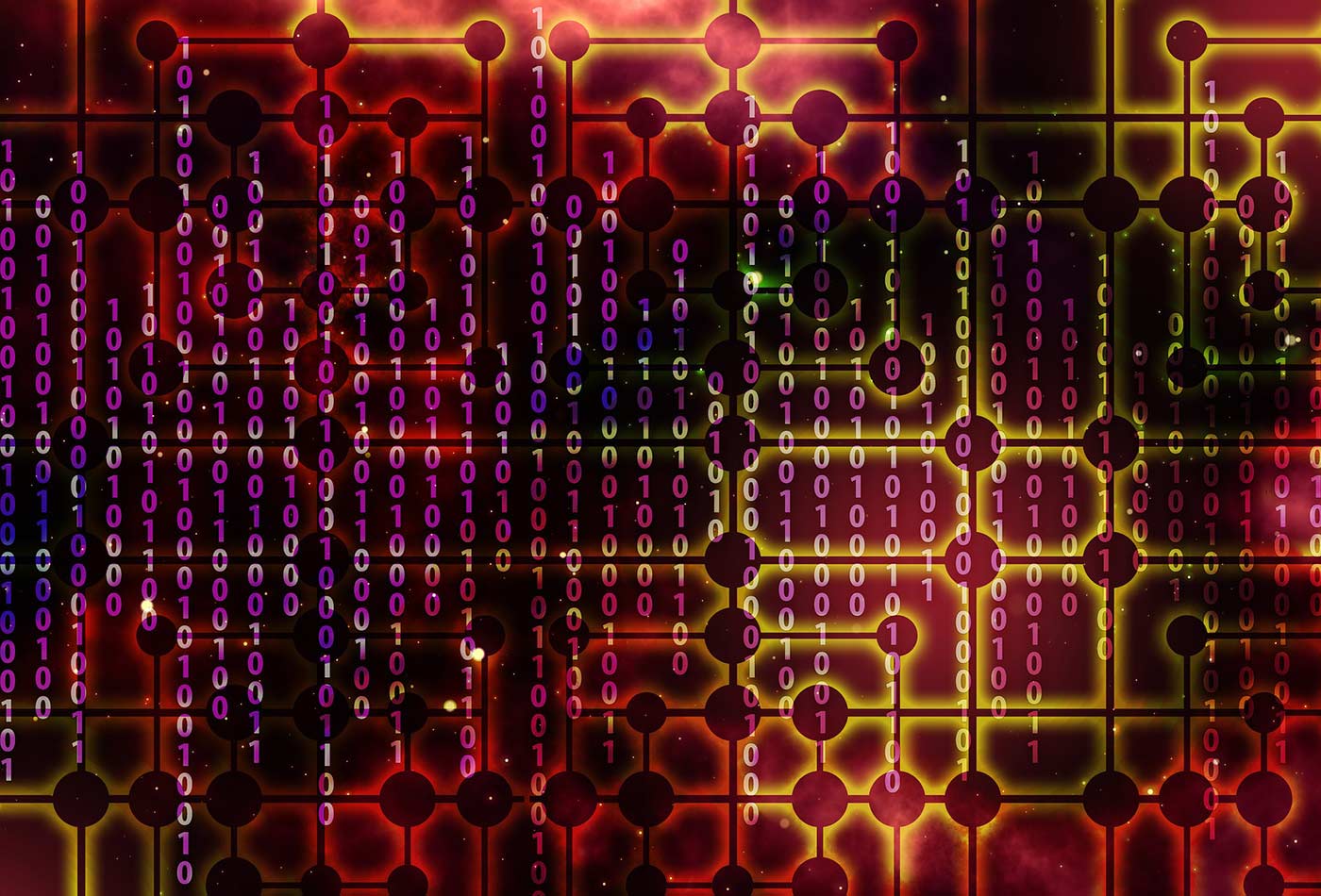
backs his claims by tracing the history of how programming has evolved, changes in programming over the past decades showed that as tools became more powerful and user-friendly, more people could create software, expanding the field rather than shrinking it.
2 — Generative AI is a Parasitic Cancer: while AI is revolutionizing certain fields, it's also wreaking destructive havoc in other places, in particular when it comes to content creation. Freya Holmér's had enough of AI generated content —in particular when it's flooding the top results of most search engines. She demonstrates this with a simple query with which she wants to learn a little more about the .gltf format, revealing that almost all of the top search results are in some form AI generated:
In the second half of the video Freya comments on the deceptive nature of this content, what's seemingly friendly sounding on the surface is simply a low effort slop that tries to compete in the search rankings, either to promote whatever product/software that website is trying to sell, or to get a few cents of ad-revenue out of your attention.
Music for Coding
This one was recommended to me while listening to Matthew Halsall's On the Go that I recommended last week — and I've pretty much just been listening to Mammal Hands this entire week, in particular their fifth studio album "Gift from the Trees" that came out last year. All tracks are really rich and atmospheric, somehow earthy and spiritual. Swirling, smooth saxophone melodies soar above complex rhythmic percussion patterns.
I can also highly recommend all other albums put out by Gondwana Records, it's all just pure ear candy!
Fun fact: after digging for the designer behind the stellar album covers, it turns out that it's actually Matthew Halsall's brother, Daniel Halsall, the creative director of Gondwana Records.
And that's a wrap — hope you've enjoyed this week's curated assortment of genart and tech shenanigans!
Now that you find yourself at the end of this Newsletter, consider forwarding it to some of your friends, or sharing it on the world wide webs—more subscribers means that I get more internet points, which in turn allows me to do more internet things!
Otherwise come and say hi over on TwiX, Mastodon, or Bluesky and since we've also got a Discord now, let me shamelessly plug it here again. If you've read this far, thanks a million! And in case you're still hungry for more generative art things, you can check out last week's issue of the newsletter here:
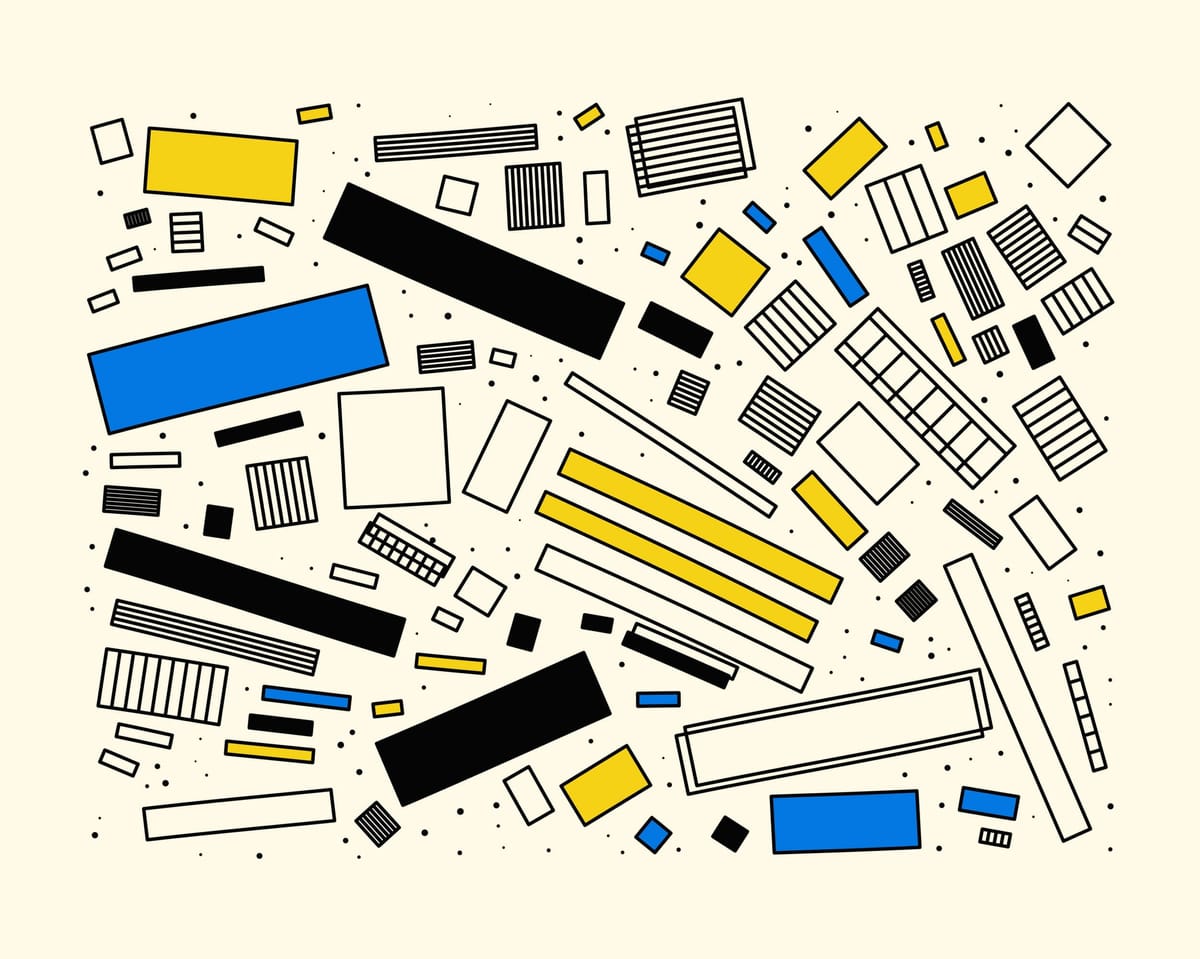
You can also find a backlog of all previous issues here:
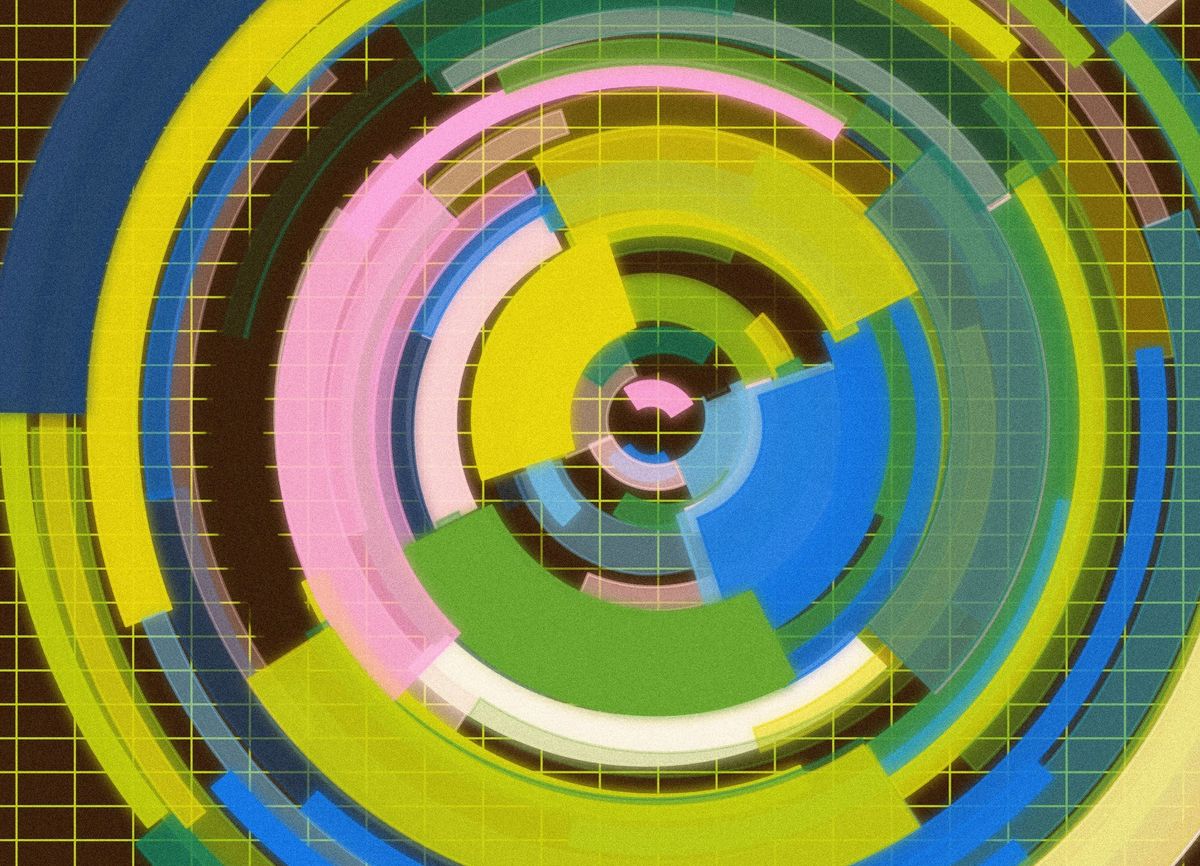
Cheers, happy coding, and again, hope that you have a fantastic week! See you in the next one!
~ Gorilla Sun 🌸